Notice
Recent Posts
Recent Comments
Link
일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | 6 | 7 |
8 | 9 | 10 | 11 | 12 | 13 | 14 |
15 | 16 | 17 | 18 | 19 | 20 | 21 |
22 | 23 | 24 | 25 | 26 | 27 | 28 |
29 | 30 |
Tags
- Colaboratory 글자 깨짐
- Python
- node.js 설치
- 타자 게임 만들기
- DB Browser
- intellij
- 모던자바스크립트
- 리액트
- Concurrently
- You are importing createRoot from "react-dom" which is not supported. You should instead import it from "react-dom/client"
- Do it 자바스크립트 + 제이쿼리 입문
- 계산맞추기 게임
- Spring-Framework
- JS 개념
- 인프런
- 따라하며 배우는 노드 리액트 기본 강의
- react
- props
- vs code 내 node
- 자바스크립트
- spring-boot
- 웹 게임을 만들며 배우는 리액트
- react오류
- 노드에 리액트 추가하기
- 거북이 대포 게임
- intllij 내 Bean을 찾지 못해서 발생하는 오류
- googleColaboratory
- 모두의 파이썬
- node.js로 로그인하기
- ReactDOM.render is no longer supported in React 18. Use createRoot instead
Archives
- Today
- Total
프로그래밍 삽질 중
Java로 계좌 관련 문제(입금, 출금, 송금 내용 포함) 본문
[문제] java로 계좌 생성 및 입금, 출금, 송금, 잔액조회, 모든 계좌 조회 만들기
1) sql문을 작성한다
2) db와 java를 연동한다
3) Account.java를 만든다
4) ① 계좌 생성 ② 입금 ③ 출금 ④ 송금 ⑤ 잔액 조회 ⑥ 모든 계좌 조회 관련 java문 만들기(Main.java)
5) 4)에서 구현한 정보들이 나오는 나머지 구현
※ 마지막에 3)의 완성본 올림
1) SQL문(테이블 이름은 account)
1
2
3
4
5
6
7
8
9
10
11
12
13
14
|
create table account (
accountId varchar2(40),
pwd varchar2(40),
name varchar2(30),
balance number);
//내용 입력하기 insert into account values('law001', 111, '강솔A', 30000);
insert into account values('law002', 222, '한준휘', 25000);
insert into account values('law003', 333, '강솔B', 50000);
commit; //commit해야 입력한 내용이 java문에서 보임
select * from account;
|
cs |
2) DB와 java문 연동(java문 이름은 Main.java)

Main.java에 들어갈 내용
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
|
String url = "jdbc:oracle:thin:@localhost:1521:xe";
String dbId= "scott";
String dbPwd = "tiger";
public static Connection getConnectivity
(String url, String dbId, String dbPwd) {
Connection conn = null;
try {
Class.forName("oracle.jdbc.driver.OracleDriver");
conn = DriverManager.getConnection(url, dbId, dbPwd);
} catch (Exception e) {
e.printStackTrace();
}
return conn;
}
|
cs |
3) Account.java
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
|
package May_0521;
public class Account {
private String accountId;
private int pwd;
private String name;
private int balance=0;
public Account(String accountId, int pwd, String name, int balance) {
/* super(); */
this.accountId = accountId;
this.pwd = pwd;
this.name = name;
this.balance = balance;
}
public String getAccountId() {
return accountId;
}
public void setAccountId(String accountId) {
this.accountId = accountId;
}
public int getPwd() {
return pwd;
}
public void setPwd(int pwd) {
this.pwd = pwd;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getBalance() {
return balance;
}
public void setBalance(int balance) {
this.balance = balance;
}
public String toString() {
return "Account [acoountid=" + accountId + ", pwd=" + pwd
+ ", name=" + name + ", balance=" + balance + "]";
}
}
|
cs |
4) ① 계좌 생성 ② 입금 ③ 출금 ④ 송금 ⑤ 잔액 조회 ⑥ 모든 계좌 조회 관련
①계좌 생성(addAccount)
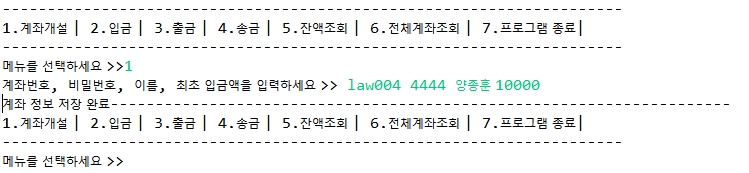
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
|
public static void addAccount(Connection conn, PreparedStatement pstmt, Account acc) {
/*private String accountId; private int pwd;
private String name; private int balance=0;*/
String sql = "insert into account values(?,?,?,?)";
int result = 0;
try {
pstmt = conn.prepareStatement(sql);
pstmt.setString(1, acc.getAccountId());
pstmt.setInt(2, acc.getPwd());
pstmt.setString(3, acc.getName());
pstmt.setInt(4, acc.getBalance());
result = pstmt.executeUpdate();
} catch(Exception e) {
e.printStackTrace();
}
System.out.print("계좌 정보 저장 완료");
}
|
cs |
② 입금(deposit, isCorrect)
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
|
public static void deposit(Connection conn,PreparedStatement pstmt, ResultSet rs ,String accountId, int money) {
String sql = null;
int balance = 0;
try {
//조회하기
sql = "select balance from account where accountId=?";
pstmt = conn.prepareStatement(sql);
pstmt.setString(1, accountId);
rs = pstmt.executeQuery();
rs.next();
balance = rs.getInt(1);
//수정하기
sql = "update account set balance=? where accountId=?";
pstmt = conn.prepareStatement(sql);
pstmt.setInt(1, balance+money);
pstmt.setString(2, accountId);
pstmt.execute();
}catch(Exception e) {
e.printStackTrace();
}System.out.println("입금이 완료되었습니다.");
}
//비밀번호 오류 시 조회 불가 ㅓ
public static boolean isCorrect(Connection conn, PreparedStatement pstmt, ResultSet rs,
String accountId, int pwd) {
String sql = "select * from account ";
boolean result = true;
try {
pstmt = conn.prepareStatement(sql);
rs = pstmt.executeQuery();
while(rs.next()) {
if(accountId.equals(rs.getString(1))&&pwd!=rs.getInt(2)) {
System.out.println("비밀번호가 일치하지 않습니다.");
result = false;
}else if(accountId.equals(rs.getString(1))&&pwd==rs.getInt(2)) {
result = true;
}
}
}catch(Exception e) {
e.printStackTrace();
}
return result;
}
|
cs |
③ 출금(withdraw)
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
|
public static void withdraw(Connection conn,PreparedStatement pstmt,ResultSet rs , String accountId, int money) {
String sql = null;
int balance = 0;
try {
//조회하기
sql = "select balance from account where accountId=?";
pstmt = conn.prepareStatement(sql);
pstmt.setString(1, accountId);
rs = pstmt.executeQuery();
rs.next();
balance = rs.getInt(1);
if(balance >= money) {
sql = "update account set balance=? where accountId=?";
pstmt = conn.prepareStatement(sql);
pstmt.setInt(1, balance-money);
pstmt.setString(2, accountId);
pstmt.execute();
System.out.println("출금이 완료되었습니다.");
}else {
//balance < money일
System.out.println("잔액이 충분하지 않습니다.");
}
}catch (Exception e) {
e.printStackTrace();
}
}
|
cs |
④ 송금(transfer)
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
|
public static void tranfer(Connection conn,PreparedStatement pstmt, ResultSet rs,
String accountId, String target ,int money) {
String sql = null;
int balance = 0;
int targetBalance;
try {
sql = "select balance from account where accountId=?";
pstmt = conn.prepareStatement(sql);
pstmt.setString(1, accountId);
rs = pstmt.executeQuery();
rs.next();
balance = rs.getInt(1);
if(balance >= money) {
sql = "select balance from account where accountId=?";
pstmt = conn.prepareStatement(sql);
pstmt.setString(1, target);
rs = pstmt.executeQuery();
rs.next();
targetBalance = rs.getInt(1);
//잔액 추가
sql = "update account set balance=? where accountId=?";
pstmt = conn.prepareStatement(sql);
pstmt.setInt(1, targetBalance+money);
pstmt.setString(2, target);
pstmt.execute();
//잔액 빠짐
sql = "update account set balance=? where accountId=?";
pstmt = conn.prepareStatement(sql);
pstmt.setInt(1, balance-money);
pstmt.setString(2, accountId);
pstmt.execute();
}else {
System.out.println("잔액이 부족합니다.");
}
}catch (Exception e) {
e.printStackTrace();
}
}
|
cs |
⑤ 잔액 조회(checkBalance)
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
|
public static int checkBalance(Connection conn, PreparedStatement pstmt, ResultSet rs, String accountId) {
String sql = null;
int balance = 0;
try {
sql = "select balance from account where accountId=?";
pstmt = conn.prepareStatement(sql);
pstmt.setString(1, accountId);
rs = pstmt.executeQuery();
rs.next();
balance = rs.getInt(1);
}catch (Exception e) {
e.printStackTrace();
}
return balance;
}
|
cs |
⑥ 전체 계좌 조회(getAllAccount)
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
|
public static List<Account> getAllAccount(Connection conn, PreparedStatement pstmt, ResultSet rs){
List<Account> aList = new ArrayList<Account>();
String sql = "select * from account";
try {
pstmt = conn.prepareStatement(sql);
rs = pstmt.executeQuery();
while(rs.next()) {
aList.add(new Account(rs.getString("accountId"), rs.getInt("pwd"),
rs.getString("name"), rs.getInt("balance")));
}
}catch(Exception e) {
e.printStackTrace();
}
return aList;
}
|
cs |
5) 4)에서 구현한 정보들이 나오는 나머지 구현
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
|
public static void main(String[] args) {
String url = "jdbc:oracle:thin:@localhost:1521:xe";
String dbId = "scott";
String dbPwd = "tiger";
Scanner scan = new Scanner(System.in); //값을 콘솔ㅔ 입력하기 위함
int select;
String accountId = null;
ResultSet rs = null;
PreparedStatement pstmt = null;
Connection conn = getConnectivity(url, dbId, dbPwd);
List<Account> aList = null;
//무한루프로 1~7번이 나오게 함 Loop:
while(true) {
System.out.println("---------------------------------------------------------------------");
System.out.println("1.계좌개설 | 2.입금 | 3.출금 | 4.송금 | 5.잔액조회 | 6.전체계좌조회 | 7.프로그램 종료|");
System.out.println("---------------------------------------------------------------------");
System.out.print("메뉴를 선택하세요 >>");
select = scan.nextInt();
switch(select) {
case 1://계좌 추가
System.out.print("계좌번호, 비밀번호, 이름, 최초 입금액을 입력하세요 >> ");
Account acc = new Account(scan.next(), scan.nextInt(), scan.next(), scan.nextInt());
addAccount(conn, pstmt, acc);
break;
case 2://입금
System.out.print("계좌번호와 입금액을 입력하세요 >> ");
deposit(conn, pstmt, rs, scan.next(), scan.nextInt());
break;
case 3://출금
System.out.print("계좌번호와, 비밀번호, 출금액을 입력하세요 >> ");
accountId = scan.next();
if(isCorrect(conn, pstmt, rs, accountId, scan.nextInt())) {
withdraw(conn, pstmt, rs, accountId, scan.nextInt());
}
break;
case 4://송금
System.out.print("계좌번호, 비밀번호, 송금받을 계좌번호, 송금액을 입력하세요 >> ");
accountId = scan.next();
if(isCorrect(conn, pstmt, rs, accountId,scan. nextInt())) {
tranfer(conn, pstmt, rs, accountId, scan.next(), scan.nextInt());
}
break;
case 5://잔액조회
System.out.print("계좌번호와 비밀번호를 입력하세요 >> ");
accountId = scan.next();
if(isCorrect(conn, pstmt, rs, accountId,scan. nextInt())) {
int balance = checkBalance(conn, pstmt, rs, accountId);
System.out.println(accountId + "의 잔액은" + balance +"원 입니다.");
}
break;
case 6://전체 계좌정보 조회
aList = getAllAccount(conn, pstmt, rs);
for(Account a : aList) {
System.out.println(a.toString());
}
break;
case 7://종료
break Loop;
}
}
}
}
|
cs |
전체 Main.java
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
119
120
121
122
123
124
125
126
127
128
129
130
131
132
133
134
135
136
137
138
139
140
141
142
143
144
145
146
147
148
149
150
151
152
153
154
155
156
157
158
159
160
161
162
163
164
165
166
167
168
169
170
171
172
173
174
175
176
177
178
179
180
181
182
183
184
185
186
187
188
189
190
191
192
193
194
195
196
197
198
199
200
201
202
203
204
205
206
207
208
209
210
211
212
213
214
215
216
217
218
219
220
221
222
223
224
225
226
227
228
229
230
231
232
233
234
235
236
237
238
239
240
241
242
243
244
245
246
247
248
249
250
251
252
253
254
255
256
257
258
259
260
261
262
263
264
265
266
267
268
269
270
271
272
273
274
275
276
277
278
279
280
281
282
283
|
package May_0521;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.util.ArrayList;
import java.util.List;
import java.util.Scanner;
public class Main {
//DB연결
String url = "jdbc:oracle:thin:@localhost:1521:xe";
String did = "scott";
String dpw = "tiger";
public static Connection getConnectivity
(String url, String dbId, String dbPwd) {
Connection conn = null;
try {
Class.forName("oracle.jdbc.driver.OracleDriver");
conn = DriverManager.getConnection(url, dbId, dbPwd);
} catch (Exception e) {
e.printStackTrace();
}
return conn;
}
//계좌 정보 DB입력 메소드
public static void addAccount(Connection conn, PreparedStatement pstmt, Account acc) {
/*private String accountId; private int pwd;
private String name; private int balance=0;*/
String sql = "insert into account values(?,?,?,?)";
int result = 0;
try {
pstmt = conn.prepareStatement(sql);
pstmt.setString(1, acc.getAccountId());
pstmt.setInt(2, acc.getPwd());
pstmt.setString(3, acc.getName());
pstmt.setInt(4, acc.getBalance());
result = pstmt.executeUpdate();
} catch(Exception e) {
e.printStackTrace();
}
System.out.print("계좌 정보 저장 완료");
}
//입금
public static void deposit(Connection conn,PreparedStatement pstmt, ResultSet rs ,String accountId, int money) {
String sql = null;
int balance = 0;
try {
//조회하기
sql = "select balance from account where accountId=?";
pstmt = conn.prepareStatement(sql);
pstmt.setString(1, accountId);
rs = pstmt.executeQuery();
rs.next();
balance = rs.getInt(1);
//수정하기
sql = "update account set balance=? where accountId=?";
pstmt = conn.prepareStatement(sql);
pstmt.setInt(1, balance+money);
pstmt.setString(2, accountId);
pstmt.execute();
}catch(Exception e) {
e.printStackTrace();
}System.out.println("입금이 완료되었습니다.");
}
public static boolean isCorrect(Connection conn, PreparedStatement pstmt, ResultSet rs,
String accountId, int pwd) {
String sql = "select * from account ";
boolean result = true;
try {
pstmt = conn.prepareStatement(sql);
rs = pstmt.executeQuery();
while(rs.next()) {
if(accountId.equals(rs.getString(1))&&pwd!=rs.getInt(2)) {
System.out.println("비밀번호가 일치하지 않습니다.");
result = false;
}else if(accountId.equals(rs.getString(1))&&pwd==rs.getInt(2)) {
result = true;
}
}
}catch(Exception e) {
e.printStackTrace();
}
return result;
}
//출금
public static void withdraw(Connection conn,PreparedStatement pstmt,ResultSet rs , String accountId, int money) {
String sql = null;
int balance = 0;
try {
//조회하기
sql = "select balance from account where accountId=?";
pstmt = conn.prepareStatement(sql);
pstmt.setString(1, accountId);
rs = pstmt.executeQuery();
rs.next();
balance = rs.getInt(1);
if(balance >= money) {
sql = "update account set balance=? where accountId=?";
pstmt = conn.prepareStatement(sql);
pstmt.setInt(1, balance-money);
pstmt.setString(2, accountId);
pstmt.execute();
System.out.println("출금이 완료되었습니다.");
}else {
System.out.println("잔액이 충분하지 않습니다.");
}
}catch (Exception e) {
e.printStackTrace();
}
}
//전달
public static void tranfer(Connection conn,PreparedStatement pstmt, ResultSet rs,
String accountId, String target ,int money) {
String sql = null;
int balance = 0;
int targetBalance;
try {
sql = "select balance from account where accountId=?";
pstmt = conn.prepareStatement(sql);
pstmt.setString(1, accountId);
rs = pstmt.executeQuery();
rs.next();
balance = rs.getInt(1);
if(balance >= money) {
sql = "select balance from account where accountId=?";
pstmt = conn.prepareStatement(sql);
pstmt.setString(1, target);
rs = pstmt.executeQuery();
rs.next();
targetBalance = rs.getInt(1);
//잔액 추가
sql = "update account set balance=? where accountId=?";
pstmt = conn.prepareStatement(sql);
pstmt.setInt(1, targetBalance+money);
pstmt.setString(2, target);
pstmt.execute();
//잔액 빠짐
sql = "update account set balance=? where accountId=?";
pstmt = conn.prepareStatement(sql);
pstmt.setInt(1, balance-money);
pstmt.setString(2, accountId);
pstmt.execute();
}else {
System.out.println("잔액이 부족합니다.");
}
}catch (Exception e) {
e.printStackTrace();
}
}
//계좌 금액 조회
public static int checkBalance(Connection conn, PreparedStatement pstmt, ResultSet rs, String accountId) {
String sql = null;
int balance = 0;
try {
sql = "select balance from account where accountId=?";
pstmt = conn.prepareStatement(sql);
pstmt.setString(1, accountId);
rs = pstmt.executeQuery();
rs.next();
balance = rs.getInt(1);
}catch (Exception e) {
e.printStackTrace();
}
return balance;
}
public static List<Account> getAllAccount(Connection conn, PreparedStatement pstmt, ResultSet rs){
List<Account> aList = new ArrayList<Account>();
String sql = "select * from account";
try {
pstmt = conn.prepareStatement(sql);
rs = pstmt.executeQuery();
while(rs.next()) {
aList.add(new Account(rs.getString("accountId"), rs.getInt("pwd"),
rs.getString("name"), rs.getInt("balance")));
}
}catch(Exception e) {
e.printStackTrace();
}
return aList;
}
public static void main(String[] args) {
String url = "jdbc:oracle:thin:@localhost:1521:xe";
String dbId = "scott";
String dbPwd = "tiger";
Scanner scan = new Scanner(System.in);
int select;
String accountId = null;;
ResultSet rs = null;
PreparedStatement pstmt = null;
Connection conn = getConnectivity(url, dbId, dbPwd);
List<Account> aList = null;
Loop:
while(true) {
System.out.println("---------------------------------------------------------------------");
System.out.println("1.계좌개설 | 2.입금 | 3.출금 | 4.송금 | 5.잔액조회 | 6.전체계좌조회 | 7.프로그램 종료|");
System.out.println("---------------------------------------------------------------------");
System.out.print("메뉴를 선택하세요 >>");
select = scan.nextInt();
switch(select) {
case 1://계좌 추가
System.out.print("계좌번호, 비밀번호, 이름, 최초 입금액을 입력하세요 >> ");
Account acc = new Account(scan.next(), scan.nextInt(), scan.next(), scan.nextInt());
addAccount(conn, pstmt, acc);
break;
case 2://입금
System.out.print("계좌번호와 입금액을 입력하세요 >> ");
deposit(conn, pstmt, rs, scan.next(), scan.nextInt());
break;
case 3://출금
System.out.print("계좌번호와, 비밀번호, 출금액을 입력하세요 >> ");
accountId = scan.next();
if(isCorrect(conn, pstmt, rs, accountId, scan.nextInt())) {
withdraw(conn, pstmt, rs, accountId, scan.nextInt());
}
break;
case 4://송금
System.out.print("계좌번호, 비밀번호, 송금받을 계좌번호, 송금액을 입력하세요 >> ");
accountId = scan.next();
if(isCorrect(conn, pstmt, rs, accountId,scan. nextInt())) {
tranfer(conn, pstmt, rs, accountId, scan.next(), scan.nextInt());
}
break;
case 5://잔액조회
System.out.print("계좌번호와 비밀번호를 입력하세요 >> ");
accountId = scan.next();
if(isCorrect(conn, pstmt, rs, accountId,scan. nextInt())) {
int balance = checkBalance(conn, pstmt, rs, accountId);
System.out.println(accountId + "의 잔액은" + balance +"원 입니다.");
}
break;
case 6://전체 계좌정보 조회
aList = getAllAccount(conn, pstmt, rs);
for(Account a : aList) {
System.out.println(a.toString());
}
break;
case 7://종료
break Loop;
}
}
}
}
|
cs |
'과거 프로그래밍 자료들 > 자바(Java)' 카테고리의 다른 글
[유튜브 강의] 소프트 캠퍼스 SpringFramework 입문2 (0) | 2021.06.03 |
---|---|
[유튜브 강의] 소프트 캠퍼스 SpringFramework 입문 (0) | 2021.05.24 |
JSP JSTL & JDBC 데이터 로딩 (0) | 2021.04.14 |
(수정 필요)JDBC&SQL를 이용해 회원 가입 폼 만들기(수정하기) (0) | 2021.04.14 |
JSP EL (Expression Language) (0) | 2021.04.13 |